HTML (HyperText Markup Language) is the backbone of web development, serving as the foundation for creating and structuring web content. Whether you’re just starting out or looking to brush up on your knowledge, being prepared for HTML-related interview questions is crucial.
In this post, I’ve categorized HTML interview questions into four key sections: Entry-Level, Mid-Level, Advanced-Level, and Scenario-Based questions. Each category is designed to test specific knowledge and skills, ensuring that you’re well-prepared no matter where you are in your journey. Let’s dive in and make sure you’re equipped with the knowledge to impress your interviewers and land that job!
ENTRY-LEVEL HTML INTERVIEW QUESTIONS
1. What is HTML and Page Structure?
HTML is the standard markup language used for creating and structuring web pages. It provides a set of tags that define the structure and content of a web page, including text, images, links and other elements. HTML documents are interpreted by web browsers to display web content.
HTML Page Structure
<!DOCTYPE html> <—- Tells version of HTML
<html> <—- HTML Root Element
<head> <—- Used to contain page HTML meta
<title>Page Title</title> <—- Title of HTML page
</head>
<body> <—- Holds content of HTML
<h2>Heading content</h2> <—- HTML heading tag
<p>Paragraph content</p> <—- HTML paragraph tag
</body>
</html>
2. What is the difference between html elements, tags , and attributes?
HTML elements are the structural components of a webpage, like <p> for paragraph. Tags denote the beginning and end of elements, like <p> and </p>. Attributes provide additional information about elements like <a href=”example.com”> where href is an attribute specifying the link’s destination.
3. What is the difference between block level elements and inline elements?
Block-level elements starts on a new line and occupy the full width available like paragraphs or headings. They create a “Block” of content.
Inline elements don’t start on a new line and only occupy the width necessary, like links or spans. They flow within the content, inline with surrounding text.
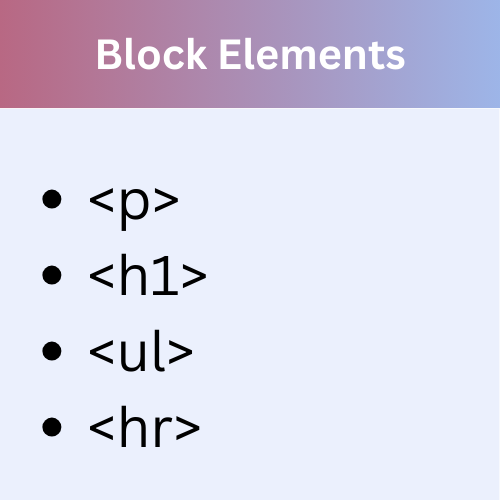
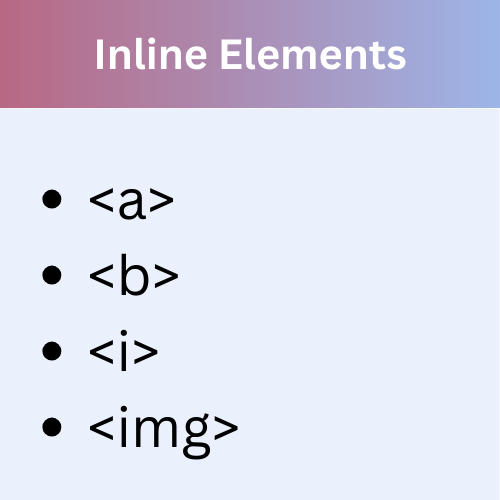
4. What are the <thead>, <tbody>, and <tfoot> tags used for?
The <thead> tag defines a set of rows that constitutes the header of a table. The <tbody> tag groups te body content of the table. The <tfoot> tag defines a set of rows that constitute the footer of a table. They help organize and structure table content in HTML.
5. What is the purpose of method attribute in the form tag?
The “method attribute” in the form tag specifies the HTTP method used to submit the form data to the server. Common values are GET and POST. “GET” appends form data to the URL, suitable for simple data retrieval. “POST” sends form data in the request body, suitable for sensitive or large data. “GET” is like putting a note on the outside of the box, and “POST” is like putting the note inside the box
6. What is the purpose of the <select> tag in HTML?
The <select> tag in HTML is used to create a drop down list of options from which the user can select one or more choices. It’s commonly used within forms to provide users with a predefined set of choices for input, such as selecting a country, a category, or a preference from a list.
<select>
<option value=”option 1″>Option 1</option>
<option value=”option 2″>Option 2</option>
<option value=”option 3″>Option 3</option>
</select>
7. What is the purpose of the <iframe> tag in HTML?
The <iframe> tag in HTML is used to embed another HTML document within the current document. It allows for the inclusion of content from another source, such as a video, map or social media widget, into a web page. This is commonly used for integrating external content while maintaining the structure of the webpage.
8. What are empty elements?
- An empty element in HTML is an element that doesn’t need content between opening and closing tags.
- Empty elements are also called a self-closing or void elements
- Empty elements in HTML are: <img>, <link>, <area>, <base>, <col>, <embed>.
9. What is the purpose of using alternative texts in images?
The purpose of using alternative texts is to define what the image is about. During an image mapping, it can be confusing and difficult to understand what hostspots correspond to aparticular linkk. These alternative texts come in action here and put a description at each link which makes it easy for users to understand the hotspot links easily.
10. How to create a nested webpage in HTML?
The HTML iframe tag is used to display a nested webpage. In other words, it represents a webpage within a webpage. The HTML <iframe> tag defines an inline frame. Forexample;
<!DOCTYPE html>
<html>
<body>
<h2>HTML Heading</h2>
<p>This is a Paragraph:</p>
<iframe src=”https://www.google.com/”height=”300″width=”400″></iframe>
</body>
</html>
11. What is the advantage of collapsing white space?
White spaces are a blank sequence of space characters, which is treated as a single space character in HTML. Because the browser collapses multiple spaces into a single space, you can indent lines of text without worrying about multiple spaces. This enables you to organize the HTML code into a much more readable format.
12. What is a marquee?
Marque is used to put the scrolling text on a web page. It scrolls the image or text up, down, left or right automatically. You should put the text which you want to scroll within the <marque>…..</marque>tag.
13. What is the use of a span tag?
The span tag is used for following thing:
For adding color on text.
For adding background on text.
Highlight any color text.
Example:
<p>
<span style=”color:#ffffff;”>
In this page we use span.
</span>
</p>
14. Why is a URL encoded in HTML?
An URL is encoded to convert non-ASCII characters into a format that can be used over the internet because a URL is sent over the Internet by using the ASCII character-set only. If a URL contains characters outside the ASCII set, the URL has to be converted. The non- ASCII characters are replaced with a “%” followed by hexadecimal digits.
15. What are HTML global attributes?
Attributes common to all HTML elements, such as id, class, style, title, and data-*.
16. Does a hyperlink only apply to text?
No, you can use hyperlinks on text and images both. The HTML anchor tag defines a hyperlink that links one page to another page. The “href” attribute is the most important attribute of the HTML anchor tag.
<a href = “…………..”> Link Text</a>
17. Do all character entities display properly on all system?
No, There are some character entities that cannot be displayed when the operating sysytem that the browser is running on does not support the characters. When that happens, these characters are displayed as boxes.
MIDDLE-LEVEL HTML INTERVIEW QUESTIONS
1. What is semantic HTML?
Semantic HTML refers to using HTML elements that convey meaning beyond just formatting to improve the structure and semantic of a webpage. By choosing appropriate tags like <header>, <footer>, <nav>, <article>, etc., it enhances accessibility, search engine optimization (SEO), and overall readability of the code and content.
2. What are Data Attributes in HTML?
- Data attributes in HTML provide a way to add custom data attributes to add additional information in elements.
- Data attributes are specified using the data- prefix.
- Data attributes can be accessed by dataset property in JS.
3. What is the canvas element in HTML5?
The <canvas> element is a container tag that is used to draw graphics on the web page using scripting language like JavaScript. It allows for dynamic and scriptable rendering of 2D shapes and bitmap images. There are several methods in canvas to draw paths, boxes, circles, text and add images, For example:
<canvas id=”myCanvas1″width=”300height=”100 style=”border.2px solid;”>
Your browser does not support the canvas element.
</canvas>
4. What is the use of figcaption tag in HTML5?
The <figcaption> element is used to provide a caption to an image. It is an optional tag and can appear before or after the content within the <figure> tag. The <figcaption> element is used with <figure> element and it can be placed as the first or last child of the <figure> element.
<figure>
<img src=”htmlpages/images/tajmahal.jpg” alt=”Taj Mahal”/>
<figcaption>Fig.1.1 – This is a tree.</figcaption>
</figure>
5. What are the HTML5 form validation attributes?
Attributes like required, pattern, min, max, step, and maxLength help validate form input.
6. Can you create a multi-colored text on a web page?
Yes. To create a multicolor text on a web page you can use <font color=”color”></font> for the specific texts you want to color.
7. What are style sheets?
Style sheets enable you to build consistent, transportable, and well-defined style templates. These templates can be linked to several different web pages, making it easy to maintain and change the look and feel of all the web pages within site.
8. What are the two types of Web Storage in HTML5?
Two storage types of HTML5 are:
Session Storage:
It stores data of current session only. It means that the data stored in session storage clear automatically when the browser is closed.
Local Storage:
Local storage is another type of HTML5 We Storage, In local storage, data is not deleted automatically when the current browser window is closed.
9. How can you optimize images for performance in HTML?
Optimizing images can be done by using formats like WebP, properly setting the srcset attribute for responsive images, using the loading=”lazy” attribute for lazy loading, and ensuring images are compressed and resized appropriately.
10. Explain the contenteditable attribute.
The contenteditable attribute makes an element editable by the user. When set to true, the content of the element can be modified directly in the browser.
<div contenteditable=”true”>This is editable text.</div>
11. How can you create a custom data attribute in HTML, and what are its benefits?
Custom data attributes are defined with the data-* prefix and can be used to store custom data private to the page or application. They are useful for accessing data via JavaScript without cluttering the HTML with non-standard attributes.
<div data-user-id=”12345″>User Content</div>
ADVANCE-LEVEL HTML INTERVIEW QUESTIONS
1. What is the purpose of the rel attribute in the <link> tag, and can you name a few values it can take?
The rel attribute specifies the relationship between the current document and the linked document/resource. Some values include stylesheet, preload, prefetch, canonical, and icon.
2. What is the <slot> element, and how is it used in web components?
The <slot> element is used in Web Components to define placeholders in a shadow DOM. These placeholders can be filled with content passed from the light DOM. It allows for customizable components.
<!– Shadow DOM –>
<template id=”my-component“>
<div class=”container”>
<slot name=”title”></slot>
<slot name=”content”></slot>
</div>
</template>
<!– Light DOM –>
<my-component>
<h1 slot=”title”>Title Here</h1>
<p slot=”content”>Content here.</p>
</my-component>
3. Describe how you can use local and session storage to manage state in a web application.
To manage state in a web application, local and session storage can be used to store data directly in the browser:
Local Storage: Stores data persistently, even after the browser is closed. Ideal for saving user preferences or settings that should be available across multiple sessions. Local storage has a larger storage capacity compared to cookies, typically around 5-10 MB per domain.
localStorage.setItem(‘theme‘, ‘dark‘);
const theme = localStorage.getItem(‘theme‘);
Session Storage: Session storage is used to store data for a single session. The data is cleared when the page session ends, i.e., when the tab or browser is closed. It’s useful for temporary data that doesn’t need to persist between sessions.
sessionStorage.setItem(‘step1Data‘, JSON.stringify(formData));
4. What are some performance considerations when using iframes in modern web developments?
Increased Load Time: Each iframe adds an extra HTTP request, which can delay the overall page load time.
Blocking Page Rendering: Iframes can prevent the parent page from rendering until the iframe content is fully loaded, potentially causing delays.
Security Concerns: Iframes can be vulnerable to security risks like cross-site scripting (XSS) attacks. Using the
sandbox
attribute helps mitigate these risks.Impact on SEO: Content within iframes is usually not indexed by search engines, which can negatively affect the SEO of the parent page.
Increased Memory Usage: Each iframe creates a separate browsing context, consuming additional memory and resources.
JavaScript Execution: Managing interactions between the parent page and iframe content can add complexity and overhead, especially with cross-origin iframes.
Limited Responsiveness: Iframes can be challenging to make responsive, requiring extra CSS and JavaScript to ensure they scale properly on different devices.
Potential Cross-Origin Issues: If the iframe loads content from a different origin, the Same-Origin Policy can limit interactions between the iframe and the parent page, requiring careful handling of cross-origin communication.
5. How does the <picture> element differ from <img> in responsive web design?
<img> Element:
- Basic Function: The
<img>
element is used to embed images in a web page. It provides a single image source. - Attributes: Commonly used with attributes like
src
,alt
,width
, andheight
. - Responsiveness: The
<img>
element alone doesn’t have built-in responsiveness. To make images responsive, you often need to use CSS techniques or media queries to adjust the image size.
<picture> Element:
- Advanced Function: The
<picture>
element is used to provide multiple image sources for different scenarios. It allows for more control over which image is displayed based on the viewport size or resolution. - Child Elements: It contains one or more
<source>
elements and an<img>
element as a fallback. The<source>
elements specify different image sources and conditions using attributes likemedia
(for media queries) andsrcset
(for different image sizes). - Responsiveness: The
<picture>
element is designed specifically for responsive images. It allows you to specify different images for different screen sizes or resolutions, improving performance and visual quality.
6. Discuss how semantic HTML can impact SEO and accessibility.
Semantic HTML uses meaningful tags (e.g., <header>
, <footer>
, <article>
, <nav>
) that convey the structure and meaning of the content. This impacts SEO and accessibility in several ways:
- SEO: Search engines use semantic tags to understand the structure and importance of content. Proper use of semantic HTML can improve a page’s ranking by making it easier for search engines to index and understand the content.
- Accessibility: Screen readers and assistive technologies rely on semantic HTML to provide context and navigation for users with disabilities. Semantic tags help these tools interpret and present the content accurately.
7. Explain the concept of Progressive Enhancement and how it applies to HTML5.
Progressive Enhancement is a strategy for web development that emphasizes providing a baseline of essential functionality for all users and progressively adding more advanced features for users with capable browsers. It ensures that a web application is accessible and functional across a wide range of devices and browsers.
- Base Functionality: Start with a simple, functional version of the site using HTML and basic CSS.
- Enhanced Features: Add enhancements such as JavaScript, advanced CSS, and other modern features for browsers that support them.
In HTML5, this approach involves using semantic elements, responsive design, and ensuring core functionality is accessible even without advanced features.
SCENARIO BASED HTML INTERVIEW QUESTIONS
1. You have a form that collects a user's profile information, including nested sections like education, work experience, and skills. How would you structure this form in HTML to maintain semantic meaning and accessibility?
To maintain semantic meaning and accessibility, you should use a combination of <fieldset> , <legend> , and appropriate form elements.
<form>
<fieldset>
<legend>Personal Information</legend>
<label for=“name”>Name:</label>
<input type=“text” id=“name” name=“name” required>
<label for=“email”>Email:</label>
<input type=“email” id=“email” name=“email” required>
</fieldset>
<fieldset>
<legend>Education</legend>
<label for=“school”>School:</label>
<input type=“text” id=“school” name=“school” required>
<label for=“degree”>Degree:</label>
<input type=“text” id=“degree” name=“degree” required>
</fieldset>
<fieldset>
<legend>Work Experience</legend>
<label for=“company”>Company:</label>
<input type=“text” id=“company” name=“company” required>
<label for=“role”>Role:</label>
<input type=“text” id=“role” name=“role” required>
</fieldset>
<input type=“submit” value=“Submit”>
</form>
This structure uses <fieldset> to group related elements, and <legend> to provide context, improving accessibility.
2. You need to optimize a web page's HTML for better SEO. What semantic elements would you use to structure the content, and why?
To optimize HTML for SEO, use semantic elements to structure content meaningfully. Key elements include:
- <header> : Wraps introductory content or navigational links.
- <nav> : Contains the main navigation links.
- <main> : Represents the dominant content of the <body>
- <article> : Encapsulates a self-contained composition.
- <section> : Groups related content together.
- <aside> : Contains content tangentially related to the content around it.
- <footer> : Contains footer information.
3. You are building a responsive website and need to ensure images are served in different sizes based on the device. How would you implement this using HTML?
Use the <picture>
element or the srcset
attribute in the <img>
tag to serve different images based on the device’s screen size.
<picture>
<source media=“(min-width: 1200px)” srcset=“image-large.jpg”>
<source media=“(min-width: 600px)” srcset=“image-medium.jpg”>
<img src=“image-small.jpg” alt=“Responsive image”>
</picture>
<img src=“image-small.jpg” srcset=“image-medium.jpg 600w, image-large.jpg 1200w” alt=“Responsive image”>
4. You need to ensure that a web page with a lot of interactive content is fully accessible. What HTML features and attributes would you use?
To enhance accessibility, use features like ARIA (Accessible Rich Internet Applications) attributes, semantic HTML, and appropriate labels.
Key practices include:
- Use aria-label, aria-labelledby, and aria-describedby for better screen reader support.
- Use <button> instead of <div> or <span> for clickable elements.
- Ensure all form elements have associated <label> tags.
- Use tabindex for managing focus order.
<button aria-label=“Close” onclick=“closeDialog()”>X</button>
<form>
<label for=“username”>Username:</label>
<input type=“text” id=“username” name=“username” required aria- describedby=“usernameHelp”>
<small id=“usernameHelp”>Enter your username here.</small>
</form>
5. Your webpage is taking too long to load due to large images and external resources. What HTML techniques would you use to optimize the loading performance?
To optimize loading performance, you can use the following HTML techniques:
- Lazy Loading Images: Use the loading=”lazy” attribute in <img> tags to delay loading images until they’re about to enter the viewport.
- Defer JavaScript Loading: Use the defer or async attribute on <script> tags to load JavaScript files asynchronously.
- Preloading Resources: Use <link rel=”preload”> to load critical resources early.
Conclusion
Preparing for an HTML interview doesn’t have to be daunting. By familiarizing yourself with the questions and answers I’ve covered, you’ll be well on your way to demonstrating your HTML expertise and confidence during your interview. Remember, practice makes perfect. Keep honing your skills, stay updated with the latest HTML developments, and you’ll be ready to tackle any challenge that comes your way. Good luck!