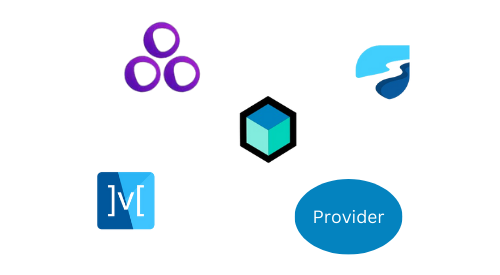
Do you find the idea of state management in Flutter a bit daunting? You’re not alone! It’s easy to feel overwhelmed when you hear phrases like “state management” and “business logic,” especially if you’re new to Flutter. But here’s the good news: state management isn’t as complex as it seems. In fact, once you get the hang of it, it’s as straightforward as pie!
In this guide, I’ll help you understand the concept of state management and walk you through choosing the best solution for your Flutter app. Whether you’re managing simple UI updates or complex app-wide data, I’ll provide clear explanations and practical examples to make state management a breeze. Let’s turn that confusion into confidence and find the perfect state management approach for your Flutter projects!
What is State Management in Flutter?
In Flutter, state refers to the data that changes over time within an app. This could include anything from the text input in a form to the visibility of a widget on the screen. State management is the practice of managing this data and ensuring that the UI updates in response to these changes. Proper state management is essential for updating and displaying the app’s state correctly in response to user interactions and other events. In Flutter, state management is crucial because it maintain app performance, simplify codebase and enhance user experience.
Why Use State Management in Flutter?
Choosing Effective state management is crucial for several reasons:
- Consistency: It ensures that the UI stays in sync with the state of your application, providing a seamless user experience.
- Performance: Proper state management helps optimize the performance of your app by minimizing unnecessary widget rebuilds and ensuring efficient state updates.
- Maintainability: It makes your code more organized and easier to maintain by separating business logic from UI code.
- Scalability: It supports building complex applications with multiple interactions and data flows, making your app easier to scale and manage.
Flutter provides several options for state management, each with its strengths and ideal use cases. Let’s check it out!
Provider
Provider is a user-friendly tool in Flutter that makes managing and sharing state across your app much simpler. It builds on top of Flutter’s InheritedWidget, which is a built-in mechanism for passing data down the widget tree. Provider helps you use this feature effectively without the need for complex boilerplate code. It allows you to easily manage and update state, ensuring your app’s data stays synchronized and accessible from different parts of your app.
Use cases: Great for managing state that needs to be accessed by multiple widgets, suitable for medium-sized apps.
import ‘package:flutter/material.dart’;
import ‘package:provider/provider.dart’;
class Counter with ChangeNotifier {
int _value = 0;
int get value => _value;
void increment() {
_value++;
notifyListeners();
}
}
void main() {
runApp(
ChangeNotifierProvider(
create: (context) => Counter(),
child: MyApp(),
),
);
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text(‘Provider Example’)),
body: Center(
child: Consumer<Counter>(
builder: (context, counter, child) => Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(‘Counter: ${counter.value}’),
ElevatedButton(
onPressed: counter.increment,
child: Text(‘Increment’),
),
],
),
),
),
),
);
}
}
Riverpod
A modern alternative to Provider, offering improved flexibility and a more robust approach to state management. It eliminates the dependency on BuildContext and provides better testability. It contains many features , enhancements, and fixes that provider doesn’t have.
Use Cases: Ideal for both small and large apps where you need more control over state and want a scalable solution.
final counterProvider = StateProvider((ref) => 0);
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return ProviderScope(
child: MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text(‘Riverpod Example’)),
body: Center(
child: Consumer(builder: (context, ref, child) {
final counter = ref.watch(counterProvider);
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(‘Counter: $counter’),
ElevatedButton(
onPressed: () => ref.read(counterProvider.notifier).state++,
child: Text(‘Increment’),
),
],
);
}),
),
),
),
);
}
}
Bloc (Business Logic Component)
A more structured approach that separates business logic from UI code. It uses Streams to manage state and is great for handling complex state and asynchronous data. It promotes immutability and has one of the best ecosysytems of supporting packages and documentation built around it.
Use Cases: Best for larger apps with complex business logic or when dealing with asynchronous operations.
import ‘package:flutter/material.dart’;
import ‘package:flutter_bloc/flutter_bloc.dart’;
// Bloc events
abstract class CounterEvent {}
class IncrementEvent extends CounterEvent {}
// Bloc state
class CounterState {
final int count;
CounterState(this.count);
}
// Bloc
class CounterBloc extends Bloc<CounterEvent, CounterState> {
CounterBloc() : super(CounterState(0));
@override
Stream<CounterState> mapEventToState(CounterEvent event) async* {
if (event is IncrementEvent) {
// Update state using emit instead of yield
emit(CounterState(state.count + 1));
}
}
}
void main() {
runApp(
BlocProvider(
create: (context) => CounterBloc(),
child: MyApp(),
),
);
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text(‘Bloc Counter Example’)),
body: CounterScreen(),
),
);
}
}
class CounterScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
BlocBuilder<CounterBloc, CounterState>(
builder: (context, state) {
return Text(‘Counter: ${state.count}’, style: TextStyle(fontSize: 24));
},
),
SizedBox(height: 20),
ElevatedButton(
onPressed: () {
context.read<CounterBloc>().add(IncrementEvent());
},
child: Text(‘Increment’),
),
],
),
);
}
}
GetX
GetX is a state management solution that integrates state management, dependency injection, and route management into a single package. It is fast, stable, light state management library in flutter. Getx syntax is easy to learn for beginners.
Use Cases: Whether you’re building a small app with a few state variables or a large application with multiple screens and interactions, GetX scales well with your app’s complexity while maintaining simplicity in your code base.
Package: https://pub.dev/packages/get
import ‘package:flutter/material.dart’;
import ‘package:get/get.dart’;
// Define the Controller
class CounterController extends GetxController {
// Observable variable
var count = 0.obs;
// Method to increment the count
void increment() => count++;
}
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
// Initialize the controller
final CounterController controller = Get.put(CounterController());
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text(‘GetX Example’)),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
// Obx widget to react to changes in the controller
Obx(() => Text(‘Count: ${controller.count}’, style: TextStyle(fontSize: 24))),
SizedBox(height: 20),
ElevatedButton(
onPressed: () => controller.increment(),
child: Text(‘Increment’),
),
],
),
),
),
);
}
}
MobX
MobX is a state management library that uses reactive programming to manage state changes. It emphasizes simplicity and efficiency, making it easy to track and react to state changes.
Use Cases: Ideal for applications where a reactive approach is preferred. Suitable for managing state with minimal boilerplate code and where automatic updates to the UI are desired.
import ‘package:flutter/material.dart’;
import ‘package:flutter_mobx/flutter_mobx.dart’;
import ‘counter_store.dart’;
part ‘counter_store.g.dart’;
class CounterStore = _CounterStore with _$CounterStore;
abstract class _CounterStore with Store {
@observable
int value = 0;
@action
void increment() {
value++;
}
}
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
final CounterStore counterStore = CounterStore();
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text(‘MobX Example’)),
body: Center(
child: Observer(
builder: (_) => Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(‘Counter: ${counterStore.value}’),
SizedBox(height: 20),
ElevatedButton(
onPressed: counterStore.increment,
child: Text(‘Increment’),
),
],
),
),
),
),
);
}
}
Which State Management is Best in Flutter?
Choosing the best state management solution in Flutter ultimately depends on your app’s specific needs and your personal preferences. For those just starting out or working on simpler projects, Provider offers a straightforward and beginner-friendly approach. If you need more flexibility and scalability, Riverpod is an excellent choice with its robust features. GetX is also excellent for its simplicity and includes features like dependency injection and route management, making it suitable for larger projects where you want more control without extra complexity.
On the other hand, Bloc provides a structured and scalable solution for large applications with complex business logic, but it may be overkill for smaller apps due to its structured approach and boilerplate code. For smaller apps, the overhead of setting up Bloc might not be necessary, and simpler solutions like Provider or GetX can be more efficient. Finally, if you prefer a reactive programming approach, MobX offers efficient state management with minimal boilerplate. Each tool has its own strengths, so consider the complexity of your app and your development style to select the most suitable state management solution.
Conclusion
Thanks for reading ! As you continue to explore Flutter, consider experimenting with different state management solutions to find the one that best fits your style and project requirements. Each tool has its own advantages, and the best choice will depend on the specific demands of your app and your development preferences. Choosing the right state management approach will set you up for success and make your Flutter development journey smoother and more enjoyable.
Pingback: Buttons in Flutter : The Ultimate Guide - learnlyzone.com